Python programming introduction
Contents
%%html
<!-- The customized css for the slides -->
<link rel="stylesheet" type="text/css" href="../../assets/styles/basic.css" />
<link rel="stylesheet" type="text/css" href="../styles/python-programming-introduction.css" />
43.1. Python programming introduction#
43.1.1. 1. What’s Python?#
Python is a high-level programming language for general-purpose programming.
Python is open-source.
Python was created by a Dutch programmer, Guido van Rossum.
The first version was released on February 20, 1991.
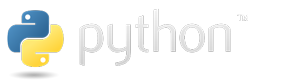
43.1.2. 2. Why Python?#
Easy to learn.
Well adopted.
Multipurpose, e.g. web development, software development, mathematics, system scripting.
Dominate data engineering and Machine Learning.
43.1.3. 3. Basic Python#
A Python script can be written in Python interactive shell or the code editor.
A Python file has an extension
.py
.
43.1.3.1. Indentation#
An indentation is a white space in a text. Indentation in many languages is used to increase code readability, however, Python uses indentation to create blocks of codes.
# incorrect
def a():
print("a")
# correct
def b():
print("b")
43.1.3.3. Data types#
43.1.3.3.1. Number#
Integer: integer(negative, zero and positive) numbers. Example: … -3, -2, -1, 0, 1, 2, 3 …
Float: decimal number. Example: … -3.5, -2.25, -1.0, 0.0, 1.1, 2.2, 3.5 …
Complex.® Example: 1 + j, 2 + 4j
43.1.3.3.2. String#
A collection of one or more characters under a single or double quote. If a string is more than one sentence then we use a triple quote.
'Asabeneh'
'Finland'
'Python'
'I love teaching'
'I hope you are enjoying the first day of Ocademy Challenge'
43.1.3.3.3. Booleans#
True # Is the light on? If it is on, then the value is True
False # I
43.1.3.3.4. List#
Python list is an ordered collection that allows to store items of different data types. A list is similar to an array in JavaScript.
Example:
[0, 1, 2, 3, 4, 5] # all are the same data types - a list of numbers
['Banana', 'Orange', 'Mango', 'Avocado'] # all the same data types - a list of strings (fruits)
['Finland','Estonia', 'Sweden','Norway'] # all the same data types - a list of strings (countries)
['Banana', 10, False, 9.81] # different data types in the list - string, integer, boolean and float
43.1.3.3.5. Dictionary#
A Python dictionary object is an unordered collection of data in a key-value pair format.
Example:
{
'first_name':'Asabeneh',
'last_name':'Yetayeh',
'country':'Finland',
'age':250,
'is_married':True,
'skills':['JS', 'React', 'Node', 'Python']
}
43.1.3.3.6. Tuple#
A tuple is an ordered collection of different data types like a list, but tuples can not be modified once they are created. They are immutable.
Example:
('Asabeneh', 'Pawel', 'Brook', 'Abraham', 'Lidiya') # Names
('Earth', 'Jupiter', 'Neptune', 'Mars', 'Venus', 'Saturn', 'Uranus', 'Mercury') # planets
43.1.3.3.7. Set#
A set is a collection of data types similar to a list and tuple. Unlike the list and the tuple, a set is not an ordered collection of items. Like in Mathematics, set in Python only stores unique items. In later sections, we will go into detail about every Python data type.
Example:
{2, 4, 3, 5}
{3.14, 9.81, 2.7} # order is not important in set
43.1.3.4. Checking data types#
To check the data type of certain data/variables we use the type
function.
type("abc")
str
type(1)
int
type([1, 2, 3])
list
43.1.3.5. Python file#
A sample Python file helloworld.py
.
print(2 + 3) # addition(+)
print(3 - 1) # subtraction(-)
print(2 * 3) # multiplication(*)
print(3 / 2) # division(/)
print(3 ** 2) # exponential(**)
print(3 % 2) # modulus(%)
print(3 // 2) # Floor division operator(//)
# Checking data types
print(type(10)) # Int
print(type(3.14)) # Float
print(type(1 + 3j)) # Complex number
print(type('Asabeneh')) # String
print(type([1, 2, 3])) # List
print(type({'name':'Asabeneh'})) # Dictionary
print(type({9.8, 3.14, 2.7})) # Set
print(type((9.8, 3.14, 2.7))) # Tuple
43.1.3.2. Comments#
Comments are very important to make the code more readable and to leave remarks in our code. Python does not run comment parts of our code.
Example: Single Line Comment
Example: Multiline Comment